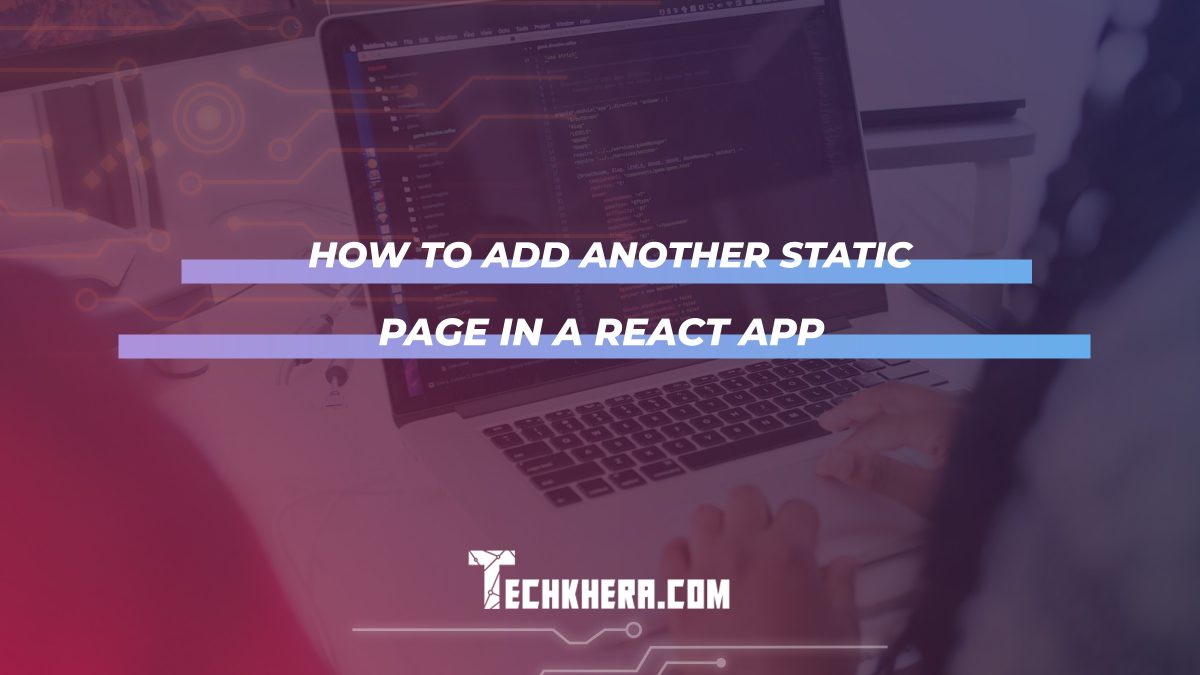
How to Add Another Static Page in a React App
Static pages play an integral role in many modern React applications. They serve as standalone components that offer non-dynamic, consistent content to users, such as an “About Us” page, a “Privacy Policy,” or even instructional content. Adding a static page in a React app might seem intimidating for beginners, but it is a straightforward process when approached systematically.
This article provides a concise step-by-step guide to show exactly how to add another static page to a React application. Whether you’re building a project from scratch or modifying an existing app, this guide has you covered.
Contents
Steps to Add a Static Page in React
1. Create a New File for the Static Page
Every React component, including a static page, typically starts with its own dedicated file. For instance, if you’d like to add an “About Us” page, create a new file in the project directory.
src/pages/About.js
Inside the newly created `About.js` file, define the structure of the static page as follows:
import React from 'react';
const About = () => {
return (
<div>
<h1>About Us</h1>
<p>Welcome to our platform! This page provides details about our journey and mission.</p>
</div>
);
};
export default About;
The above code sets up a simple static page with a heading and a paragraph. You can start by populating this file with additional static data as necessary.
2. Configure Routing for the Static Page
React apps commonly use React Router for navigation. To incorporate your new static page into the app’s routing, you’ll need to update an existing router configuration.
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
const App = () => {
return (
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</Router>
);
};
export default App;
The above snippet adds a new route for the “About Us” page (`/about`). When users navigate to that URL, the corresponding static page will render seamlessly.
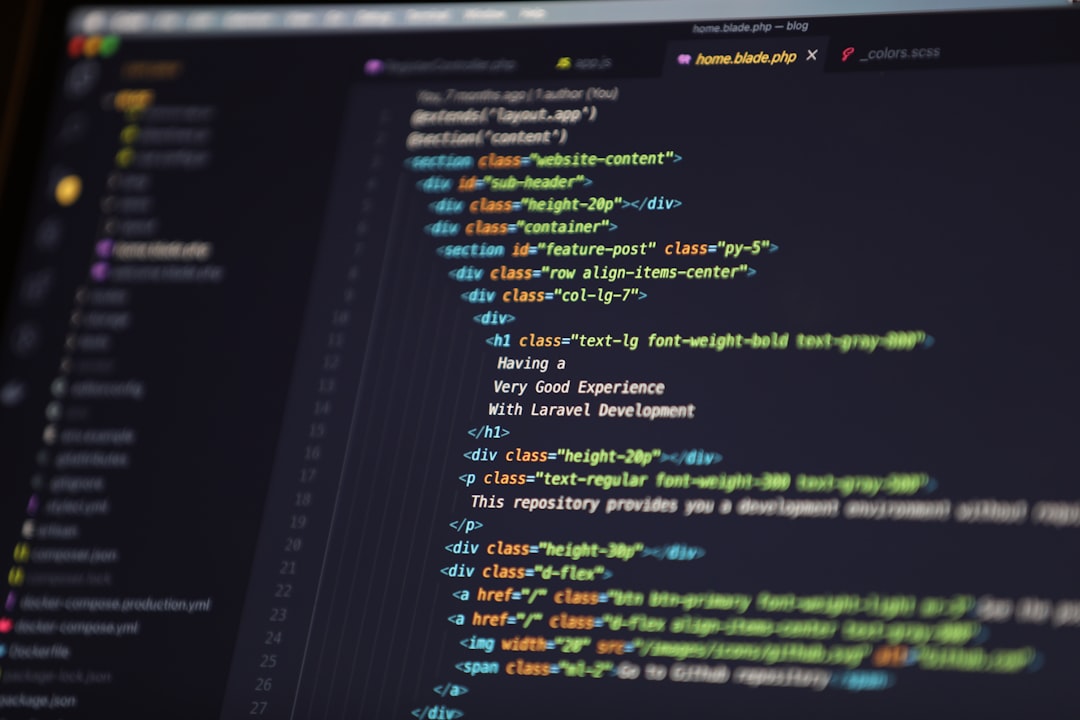
To ensure ease of access for users, a new static page should be integrated into your application’s navigation menu. Open your navigation component (e.g., `Navbar.js`) and add a link to the “About” page.
import React from 'react';
import { Link } from 'react-router-dom';
const Navbar = () => {
return (
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About Us</Link></li>
</ul>
</nav>
);
};
export default Navbar;
This addition ensures that users can navigate to the new static page directly from the main navigation menu.
4. Test the Application
After adding and configuring the static page, launch your application using:
npm start
Navigate to the URL path (e.g., `http://localhost:3000/about`) to verify that the static page renders correctly. Additionally, try using the navigation link in your app to confirm that routing between pages functions as intended.
5. Customize and Style the Static Page
Static pages should not only contain the right content but should also align visually with the rest of the application. You can use CSS or a library like Tailwind CSS to add styles to the page. For example, to apply basic styles, create a CSS module or update global stylesheets as necessary.
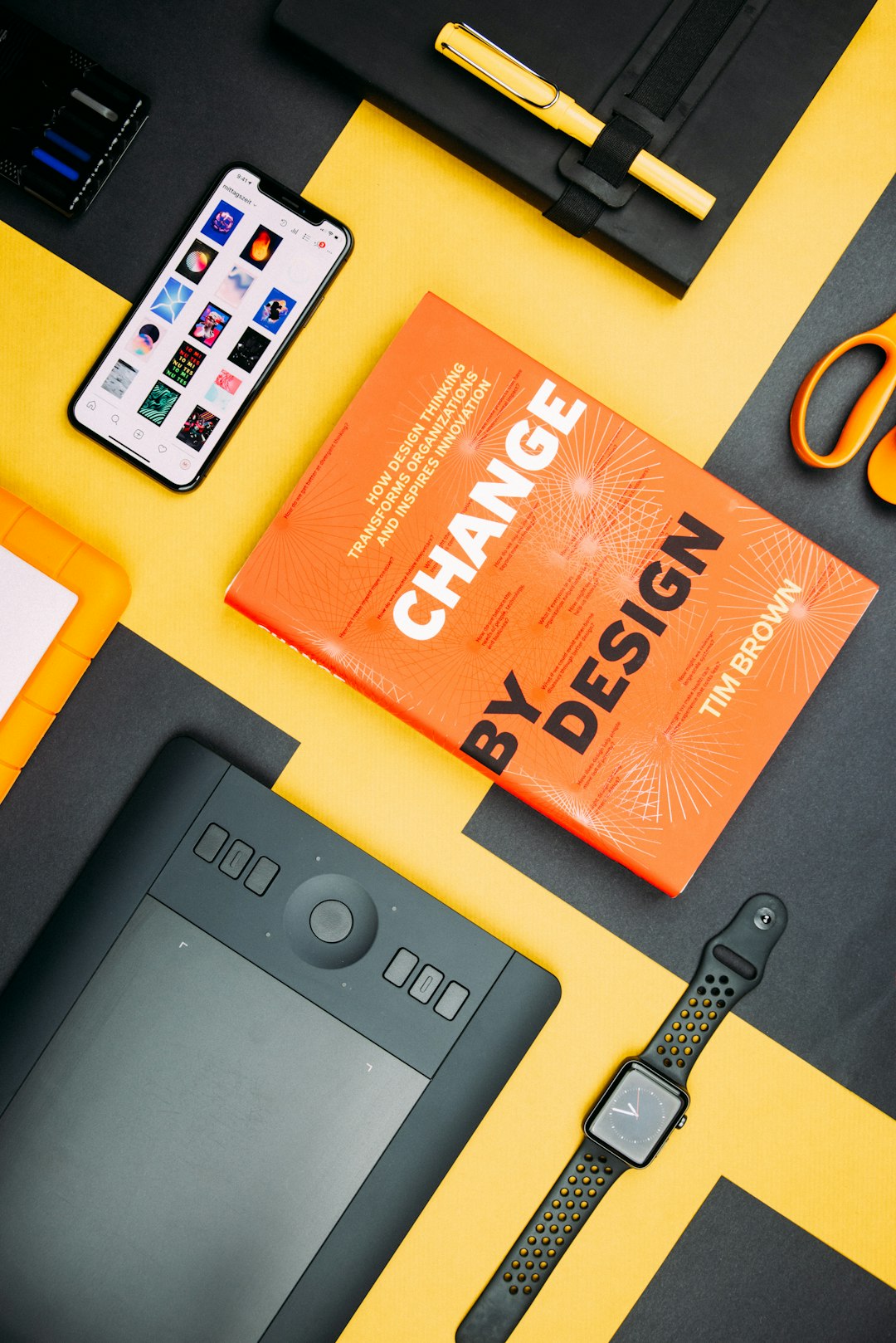
Here’s an example of basic styling using a CSS module:
import React from 'react';
import styles from './About.module.css';
const About = () => {
return (
<div className={styles.container}>
<h1 className={styles.heading}>About Us</h1>
<p className={styles.text}>We are passionate about delivering solutions for our users.</p>
</div>
);
};
export default About;
Pairing the right visual design with meaningful content is essential to creating a compelling user experience.
FAQs
- Q: Can I create multiple static pages in a React app?
A: Absolutely. Simply repeat the process outlined above for each additional page you want to add. - Q: How do I make a static page responsive?
A: Utilize CSS media queries or responsive design libraries like Bootstrap or Tailwind CSS to optimize your static page for various screen sizes. - Q: Do I need React Router for static pages?
A: While React Router simplifies navigation, it is possible to create static pages without it. However, you’ll need to handle navigation and route changes manually. - Q: Can static pages include external resources?
A: Yes, static pages can include external assets like images, videos, or stylesheets, provided they are correctly imported and referenced within the component.
With this guide, adding a static page to a React app becomes a smooth process. By following these simple steps, developers can expand their applications with minimal hassle while maintaining a scalable structure.