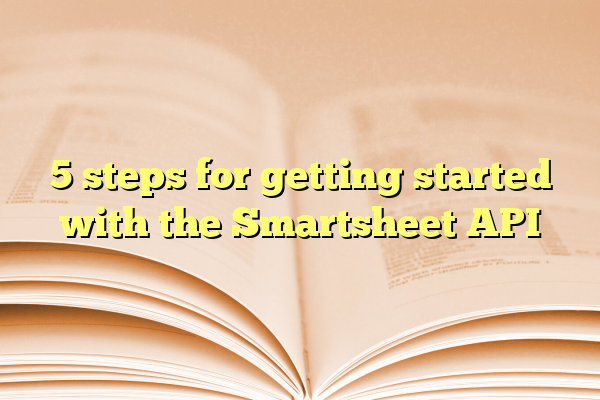
5 steps for getting started with the Smartsheet API
Smartsheet is a powerful tool for managing projects and data. But did you know it also has an API? With the Smartsheet API, you can automate tasks, integrate with other apps, and customize workflows.
If you’re new to APIs, don’t worry! This guide will walk you through five simple steps to get started.
Contents
1. Get Your API Key
Before you can use the Smartsheet API, you need an API key. This key allows your applications to communicate with Smartsheet.
- Log in to your Smartsheet account.
- Go to Account > Apps & Integrations.
- Find the API Access section.
- Click Generate New Access Token.
- Copy and save your token securely.
This key is like a password, so keep it private!
2. Understand the API Documentation
The Smartsheet API has a detailed documentation. It explains everything the API can do.
Here’s what you’ll find:
- How to authenticate using your API key
- How to read and write data
- Common API request formats
- Examples of real API responses
Take some time to browse. Understanding the docs will save you hours of guessing!
3. Make Your First API Request
The best way to learn is by doing! Let’s start with a simple request to list all sheets in your Smartsheet account.
Using cURL
curl -X GET "https://api.smartsheet.com/2.0/sheets" \
-H "Authorization: Bearer YOUR_API_KEY"
This sends a request to Smartsheet’s API asking for a list of all sheets.
Using Python
import requests
api_key = "YOUR_API_KEY"
headers = {"Authorization": f"Bearer {api_key}"}
response = requests.get("https://api.smartsheet.com/2.0/sheets", headers=headers)
print(response.json())
Run this in Python, and you’ll see a response with your sheet data!
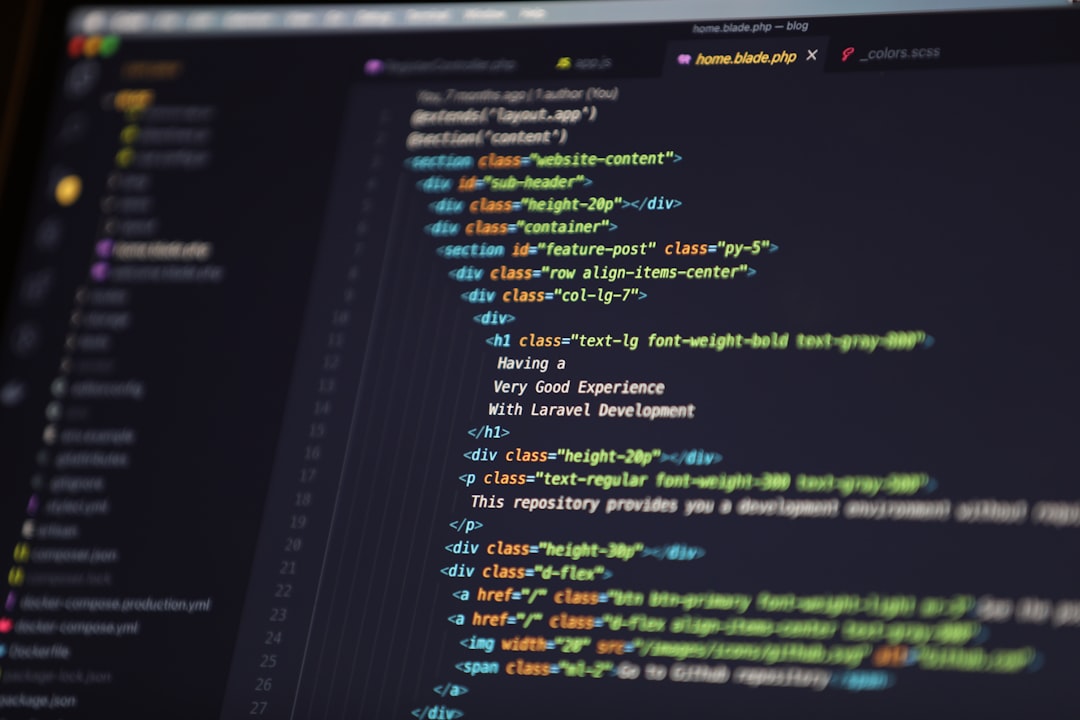
4. Create a New Sheet
Now, let’s try creating a new sheet with the API.
- Use the
POST
method. - Send JSON data with the sheet’s name and columns.
Example Request:
curl -X POST "https://api.smartsheet.com/2.0/sheets" \
-H "Authorization: Bearer YOUR_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"name": "My First API Sheet",
"columns": [
{"title": "Task Name", "type": "TEXT_NUMBER"},
{"title": "Due Date", "type": "DATE"},
{"title": "Status", "type": "PICKLIST", "options": ["Not Started", "In Progress", "Complete"]}
]
}'
If successful, you’ll get a response with the new sheet ID!
5. Update and Delete Data
APIs don’t just read and create data. You can also update and delete information.
Updating Rows:
To update a row, send a PUT
request with new data, including the sheet ID and row ID.
Deleting a Sheet:
To delete a sheet, send this request:
curl -X DELETE "https://api.smartsheet.com/2.0/sheets/SHEET_ID" \
-H "Authorization: Bearer YOUR_API_KEY"
Replace SHEET_ID
with the actual sheet ID.
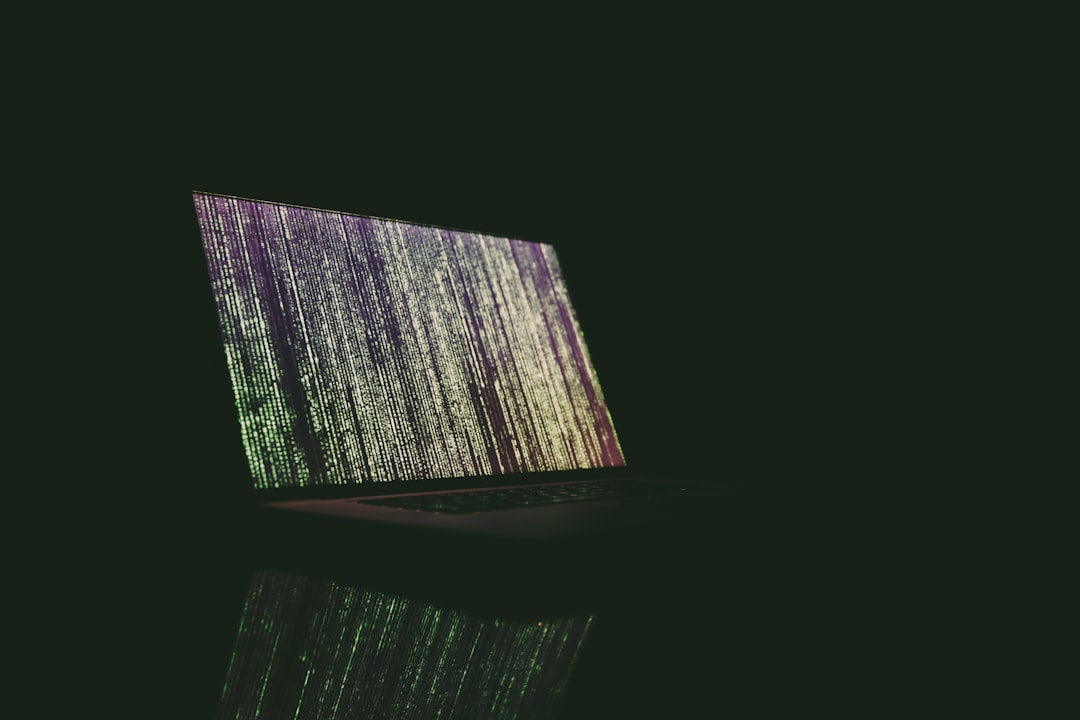
You’re Ready to Build!
That’s it! You’ve learned how to:
- Generate an API key
- Read the API documentation
- Make API requests
- Create a sheet
- Update and delete data
Now, try automating your workflows with the Smartsheet API. Experiment, build, and have fun! 🚀